Node.js vs Swift basically comes down to what you’re aiming for. Node runs JavaScript on the server in a non-blocking way, so it just keeps handling incoming stuff without freezing up. That’s why it’s awesome for chat systems, live scores, or any scenario with tons of simultaneous connections. Swift, on the other hand, is Apple’s language for iOS and macOS. If you need a native iPhone app, Swift is where you go. It’s compiled, feels strict at times, but avoids a lot of random runtime bugs.
No point forcing Swift on a real-time backend, or Node on an iOS app. Just use what fits.
Node.js in Action
Imagine you’re building an app that pushes updates the instant they happen — like chat, or live scoreboards, or even collaborative document editing. You need speed, lots of ongoing connections, and no noticeable lag. Node is pretty much made for that. It’s built around non-blocking I/O and WebSockets, so you can handle thousands of open sockets without everything grinding to a halt.
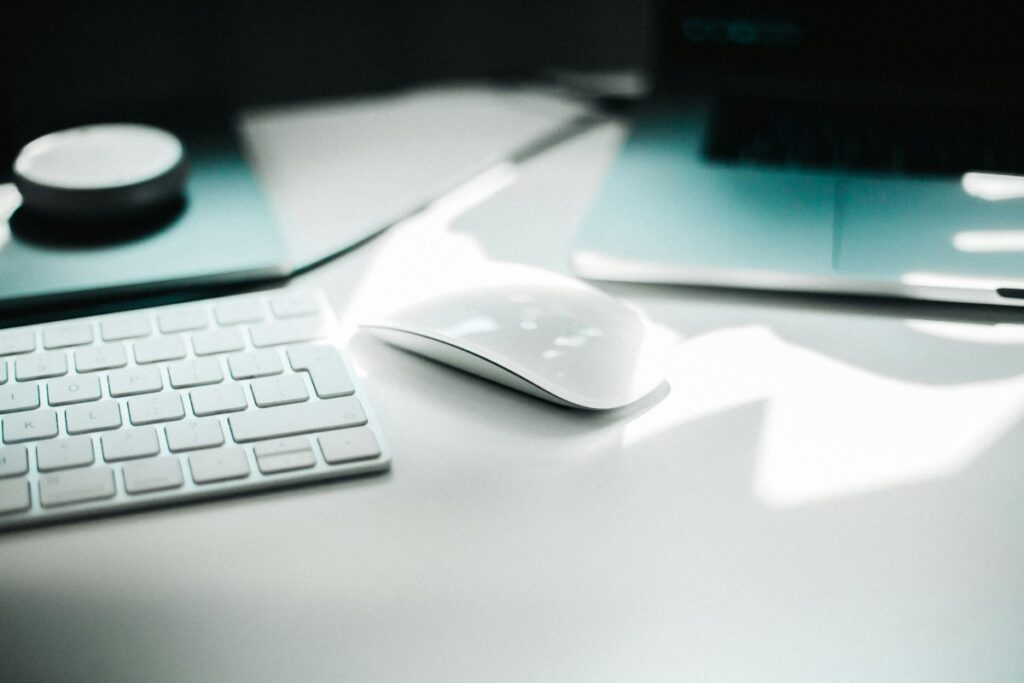
Here’s a bare-bones WebSocket example:
javascript
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
ws.on('message', (message) => {
console.log('received:', message);
});
ws.send('Hello! Message From Server');
});
What’s happening? A user connects, the server listens, and when someone sends a message, it just prints it to the console. Then it sends back a greeting. Simple, but it shows off Node’s real strength — staying connected and responsive.
Traditional servers can bog down if too many people jump on at once. Node usually doesn’t. It’s designed to handle real-time features like chats or dashboards without breaking a sweat. The main learning curve is the async nature. If you’re used to a typical “do this, then do that” flow, promises and callbacks might feel strange. But once it clicks, you’ll wonder how you ever got by with blocking code.
Your app doesn’t stop in its tracks for one request. It just keeps handling new ones. Whenever an I/O operation finishes, Node picks back up where it left off. That’s the entire point. It’s event-driven and doesn’t block, so you can manage a bunch of connections efficiently.
Overall, if you want to build a real-time service that handles a ton of concurrent connections, Node’s a great pick. But if you’re building something that needs native iOS performance or taps into Apple’s frameworks, Swift is the better route. Know your project, know your tools, and pick whichever solves your problem with fewer headaches.
Swift
Swift is Apple’s go-to for building apps on iOS and macOS — and for good reason. The syntax is clean, easy to read, and you get strong type safety baked in. That means fewer surprises when you run your code — a lot of bugs get caught before you even hit “Build.”
Everything in Swift — variables, functions, even optionals — goes through strict checks. The compiler doesn’t let much slide, which is a pain sometimes, but it saves you from weird edge cases later.
Here’s a simple example that shows how Swift handles safety and structure without feeling heavy:
import Combine
let publisher = Just("Hello, Swift!")
.sink { value in
print(value)
}
Swift isn’t just about speed — though it’s fast. It compiles down to native code, so animations are smooth, app launch times are quick, and you’re working directly with the hardware. But what really makes Swift click for iOS devs is how naturally it fits with Apple’s stack.
With Combine, Swift handles reactive programming in a clean way — data streams, user input, async events — all flow through structured pipelines. It’s a solid choice if you’re building something that needs to respond to changes in real time without becoming a mess of callbacks.
The syntax is expressive but tight. You get modern features — optionals, pattern matching, concise closures — without losing control over memory or performance. It’s readable, but still strict enough to prevent you from doing dumb stuff by accident.
And then there’s the tooling. Xcode is solid, especially when paired with Playgrounds, which let you try things out without spinning up a full project. Pair Swift with SwiftUI or UIKit, and you’ve got a workflow that feels fast and pretty enjoyable once you’re in the groove.
Outside iOS? Swift’s making moves. Server-side Swift is real — with frameworks like Vapor — but let’s be honest: it hasn’t caught on like Node has in the back-end world. It works, it’s clean, but the community and ecosystem are smaller.
Divergent Philosophies
Node.js comes from the web world — built on JavaScript, designed to be fast, flexible, and non-blocking. It runs on an event loop and handles huge amounts of concurrent traffic without slowing down. If you’re dealing with lots of requests or real-time streams, Node’s built for it.
Swift, by contrast, was Apple’s answer to the complexity of Objective-C. It’s strong on safety, type enforcement, and performance. It compiles straight to native code, which means it runs fast on Apple hardware and makes full use of system resources.
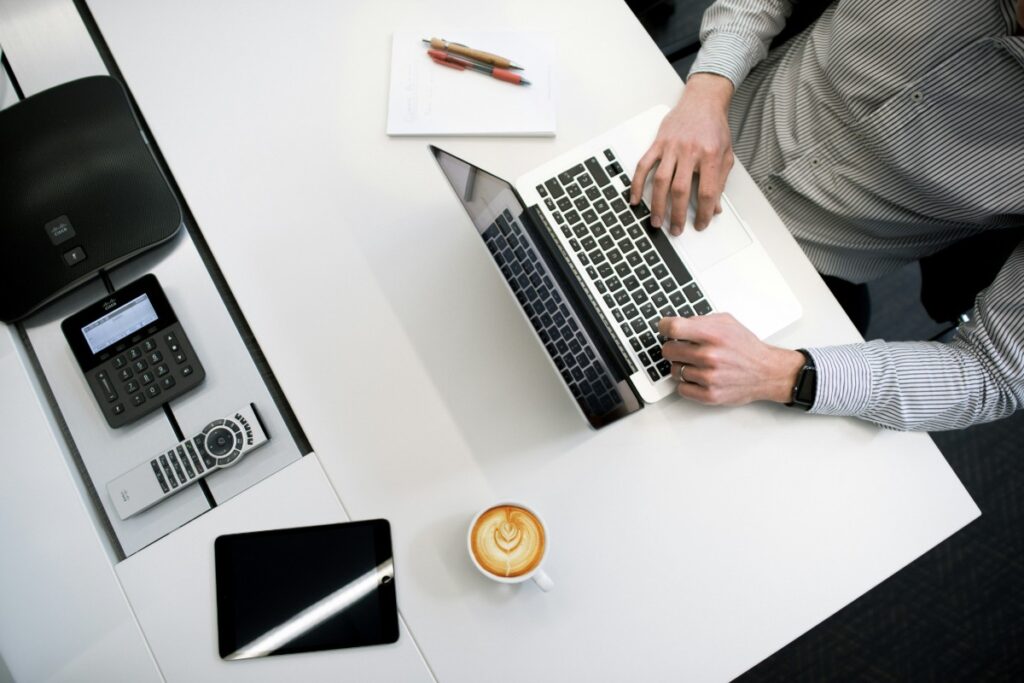
Node thrives on the back end, but because it’s JavaScript, it plays well with the front end — same language, shared models, smooth handoffs. That’s a huge plus for teams building full-stack apps.
Swift’s in its own lane. Built for Apple gear — and it shows. You can run it on the server with Vapor or similar, but let’s be honest, most people aren’t doing that. Server-side’s still Node’s game.
Different tools. Pick based on what you’re building, not what’s trendy.
Performance Benchmarks
Node.js vs Swift — Different Worlds, Different Needs
Node came out of the need to make JavaScript work on the server. It’s fast, it doesn’t block, and it can juggle a ton of things at once. It’s perfect for apps that need to stay open and responsive — chat servers, APIs, anything where real-time matters.
Swift started because Apple needed to move on from Objective-C. Too many sharp edges, too much room for bugs. Swift cleans that up — it’s strict, typed, and fast. Apps compile down to native machine code, so they run smoothly on iPhones without a ton of overhead.
Node’s a natural fit if you’re doing full-stack JavaScript. You can share models between front and back end, keep everything in one language, and scale out easily.
Swift isn’t trying to be everything. It’s built for Apple devices — and it’s very good at that. Yes, you can run Swift on the server with tools like Vapor, but that’s still a smaller scene. Most people reach for Swift when they need tight, fast mobile apps that feel native because they are.
They’re just different tools for different jobs. It’s not really a debate — it’s more about picking what fits.
const http = require('http');
http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello World\n');
}).listen(3000);
console.log('Server running at http://localhost:3000/');
Node’s designed to handle constant incoming requests without blocking. It runs everything through an event loop, so no single request ever freezes the system. That makes it perfect for high-traffic environments. Throw in Docker or Kubernetes, and it scales out easily — spin up more containers, handle more load, no drama.
Swift can run on the back end — frameworks like Vapor make it possible — but it’s not common in large-scale clusters. Part of that is ecosystem: Node’s been around longer, has a huge community, and battle-tested patterns for scaling. Swift’s server-side tools just aren’t as mature, and most teams building distributed systems stick with what’s already proven.
Ecosystem and Library Support
Libraries can make or break your workflow. The stronger the ecosystem, the less time you spend reinventing the wheel.
Node.js
- The npm ecosystem is huge — you’ll find packages for just about anything: auth, routing, testing, data viz, you name it.
- Express is the go-to for quick, clean API setup. It handles routing, middleware, and basic server logic with minimal fuss.
- Socket.io makes real-time features easy to wire up — perfect for chat apps, multiplayer games, or live dashboards.
- And since it’s all JavaScript, you can share code between the front end and back end — less mental overhead, fewer bugs.
Swift
- Owns the Apple ecosystem — iOS, macOS, watchOS, tvOS.
- Uses libraries like Alamofire for network calls, SwiftyJSON for clean JSON parsing, and Combine for reactive workflows.
- Works beautifully with SwiftUI, which powers clean, modern UIs on Apple devices.
- On the server side, tools like Vapor exist, but the community and library support still lag behind Node — especially outside Apple platforms.
Here’s a quick Express config to show just how simple it is to get an API up and running:
const express = require('express');
const app = express();
app.use(express.json());
app.post('/api/data', (req, res) => {
res.status(201).send(req.body);
});
app.listen(3000, () => {
console.log('Express server running on port 3000');
});
The Express snippet above shows exactly why Node is so popular: quick setup, easy handling of JSON, and barely any boilerplate. You can wire it into other services or databases without jumping through hoops. Swift frameworks like Vapor are moving in that direction, but Node still leads when it comes to back-end adoption and ecosystem maturity.
Learning Curves and Development Speed
Node.js
- If you’ve written JavaScript in the browser, you’re already halfway there. Node uses the same language, so jumping into the back end feels familiar.
- The tricky part? Async behavior. New devs often expect things to run top to bottom. With callbacks, promises, and now async/await, it takes a minute to adjust.
- That said, prototyping is fast. Thanks to npm, you can get an API or tool running with a few lines of code.
- And since both front-end and back-end use JavaScript, you can share validation logic, types (with TypeScript), or even utility functions across the stack.
Swift
- It’s much friendlier than Objective-C ever was, but it still has rules. You’ve got to deal with optionals, strong typing, and strict compiler checks.
- But those same rules help avoid runtime crashes and memory issues. Swift is strict for a reason.
- Xcode is a big win here — live previews, UI tools, auto-generated tests — it’s all built in.
- Swift’s async/concurrency tools have improved a lot, but server-side Swift still isn’t where Node is in terms of distributed system support or production adoption.
Here’s a small snippet to show what async looks like in Node using async/await:
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
};
fetchData();
And here, a Swift function harnesses optionals:
func fetchUserID(identifier: String?) {
guard let id = identifier else {
print("Invalid identifier")
return
}
print("User ID: \(id)")
}
Use Cases and Technology Alignment
- Node.js
- Dominates real-time chat infrastructures, gaming frameworks, or multi-user back-ends that crave immediate data flux.
- Bolsters cross-platform aspirations through JavaScript’s universal presence.
- Pairs well with microservices, orchestrating them with minimal overhead.
- Swift
- Excels in performance-critical iOS applications, especially those with elaborate interfaces and animations.
- Strong typing deflects many runtime failures before they arise.
- Delivers synergy with Apple’s native toolkit, including CloudKit and Core Data.
- Leverages SwiftUI for streamlined, declarative UI design.
Below is a Node.js WebSocket setup:
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', ws => {
ws.on('message', message => {
console.log('received: %s', message);
ws
Node’s event-driven model makes it a natural fit for stuff like real-time collaboration, streaming, or handling live sensor data. It keeps latency low, even with lots of simultaneous users.
On the other side, Swift shines when you’re building tight, responsive iOS apps. Its direct access to system features — like the camera, haptics, or GPU rendering — lets you build stuff that feels smooth and native. Apple’s frameworks are built to take full advantage of it.
Scalability and Maturity
Scalability
Node.js vs Swift comes into focus when you start thinking about scale. Node is built for it. You can run it in containers, spin up multiple instances, and throw it behind a load balancer. With its non-blocking nature, each process can handle tons of connections — ideal for APIs, real-time services, or microservices at scale.
Swift can scale on the server, and there are teams doing it, but it’s less common. A lot of Swift use cases are tied directly to iOS apps — where massive server clusters aren’t usually needed. If you are using Swift on the back end for something big, it’s probably part of a more niche stack.
When it comes to orchestration with tools like Docker or Kubernetes, Node has a clear lead. It’s been used that way for years, and the ecosystem around it is built for that kind of scale.
Maturity
Node’s been around long enough that most of the kinks are worked out. There’s a package for nearly everything, and if something breaks, odds are someone else has already run into it — and fixed it.
Swift is rock solid on Apple devices. But in the back-end world, it’s still growing. Vapor and other server-side frameworks are improving, but it’s not as plug-and-play. You’ll probably need someone who knows Swift really well to run and maintain a production Swift server. Node, on the other hand, has a massive pool of experienced devs and mature tooling around it.
Decision Factors
- Team Expertise
If your team’s already strong in JavaScript, Node.js is the obvious pick. You can hit the ground running.
If you’ve got iOS devs who know Swift inside out, leaning into Swift — especially for iOS-first products — makes sense.
- Project Scale
Node scales easily. You can containerize it, deploy it serverless, spin up clusters — all with mature tooling.
Swift can scale too, but the setup’s less common and needs more custom work, especially for distributed systems.
- User Experience
Need a smooth, native iOS interface? Swift is the clear winner. SwiftUI and UIKit are built for that level of polish.
Node isn’t about UI — it powers what runs behind the scenes and integrates well across platforms.
- Deployment
Node.js runs anywhere — most cloud providers support it out of the box.
Swift works on Linux, and frameworks like Vapor help, but it’s still not as widely supported or battle-tested on most hosting platforms.
- Maintenance and Updates
Node moves fast, with tons of updates, libraries, and community support.
Swift is solid, especially on Apple hardware, but OS-level changes and device constraints can affect timelines and compatibility.
If you’re targeting iPhones and want things to feel native — smooth animations, deep hardware integration, minimal battery hit — Swift is the way. That’s what it’s made for. It plays nice with Apple’s stack, and it feels tight. Not always fast to build, but very clean when you get it right.
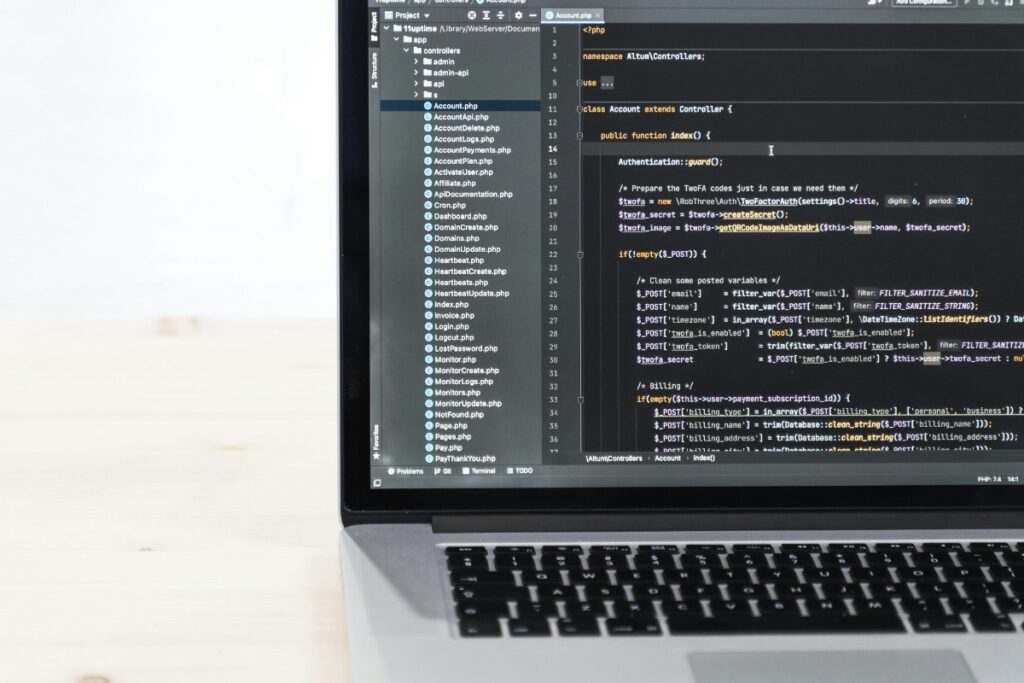
Node’s a different beast. It’s great if you’re working across platforms, dealing with lots of connections, or building something real-time. It’s lightweight, flexible, and you can move fast — especially if your team already knows JavaScript.
Swift can do backend stuff now, sure, but let’s be real — most people still reach for Node when they need to scale APIs or handle a ton of traffic.
So yeah — it comes down to your use case and your team. There’s no right answer across the board. Just pick the one that makes sense and don’t try to force it.