1. Introduction to serverless architecture
Serverless architecture (often called Functions as a Service, or FaaS) flips the old way of hosting on its head. Sure, there are still physical servers, but you never deal with them. The cloud handles all the gritty stuff — CPU provisioning, memory limits, OS updates, you name it. If you need a closer look at how it all works behind the scenes, a Serverless Architecture Deep Dive can shed more light on the specifics.
You just write the function. Some event triggers it (maybe an API call, maybe a file upload), it runs, and then it’s gone. You pay for the actual run time instead of keeping a server up round the clock.
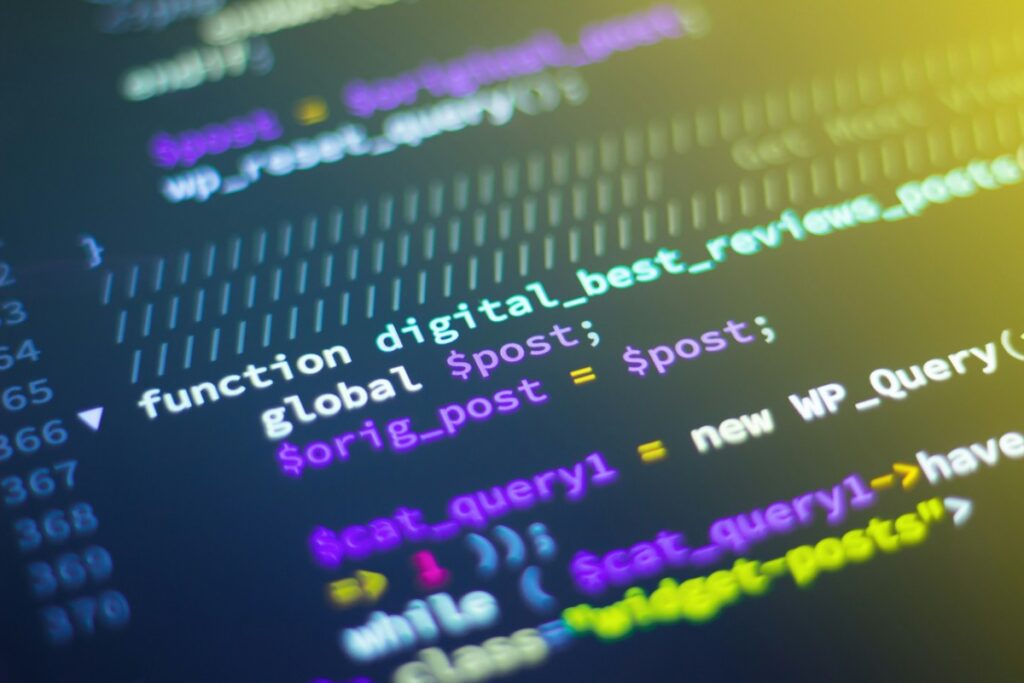
AWS Lambda, Azure Functions, and Google Cloud Functions are the big ones. They’re built to do things like webhooks, lightweight APIs, or quick background jobs. They spin up fast and shut down just as quickly.
Serverless: why people use it
- It scales automatically, no fiddling.
- You only pay when the code’s running.
- You don’t have to babysit servers or patch VMs.
It’s not perfect for everything, but for small bursts of work or fast prototyping, it’s a no-brainer.
2. Core concept and paradigm shift
Most hosting models keep servers running 24/7, even if no one’s using them. Serverless is different: nothing runs until something triggers it. If a request shows up or someone uploads a file, then your function spins up to handle that and shuts down afterward.
No more setting up entire servers. No patching, no capacity planning, no wasted resources. Instead, you write small, event-driven bits of code. Need an image resized? That’s one function. Need an email sent? That’s another. You pay only for when these things actually do something.
If ten people hit your function at once, ten instances fire up automatically. If nobody hits it, you’re paying zero. It’s lean and saves you from headaches.
3. Key serverless components
There are four main pieces to a typical serverless setup:
- FaaS (Functions as a Service): Like AWS Lambda. You write tiny bits of code that run when events happen.
- BaaS (Backend as a Service): Something like Firebase. It handles stuff like authentication, databases, file storage — all managed for you.
- API gateway: This is the traffic cop if you expose functions over HTTP. It handles routing, rate limits, CORS, etc.
- Workflow tools: For chaining multiple steps. AWS Step Functions is a classic example, so you don’t need to manage states or complex logic in your own code.
Example: minimal AWS Lambda
javascript
CopyEdit
exports.handler = async (event) => {
const name = event.queryStringParameters.name || ‘World’;
return {
statusCode: 200,
body: JSON.stringify(`Hello, ${name}!`),
};
};
An HTTP request comes in, Lambda runs, returns a greeting. No server, no manual environment. Perfect for quick tasks.
4. Cost efficiency and pay-as-you-go model
In serverless, you’re billed by how many times your function gets called and how long it runs. If nobody calls it, you pay nothing. Compare that to a traditional server that racks up costs whether it’s busy or not.
If traffic spikes, the system spins up more function instances automatically. You’re never guessing how many servers to rent. It’s straightforward: pay for code execution, skip the overhead.
5. Built-in scalability
Serverless practically defines automatic scaling. You don’t configure auto-scaling groups or load balancers. The platform does it. A traffic spike spins up more instances; a lull spins them down.
You do have to watch out for surprise costs if there’s an unexpected surge in calls, because each invocation is billed.
5.1 How auto-scaling actually works
Under the hood, new containers or micro-VMs handle extra requests. You don’t see or touch them. Your job is just to make sure the function code is efficient enough.
5.2 The cold start problem
If a function’s been idle, the first request can be slow while the environment boots up. That might not matter if your app isn’t super time-sensitive, but it’s something to keep in mind.
You can keep functions “warm” by pinging them, but that adds a tiny cost and complicates the purely on-demand approach.
6. Integration with third-party services
Serverless functions rarely live alone. They often tie into databases, storage, or notification systems through APIs or SDKs. That means you don’t stand up separate servers for logging, analytics, or file management — you just call a service. It simplifies your stack and shortens your to-do list.
7. Node.js vs. Swift: strategic technology considerations
Which language you pick depends on your use case and your team. Node.js is super popular for async tasks, huge library support, and quick development. It also integrates seamlessly with most serverless providers.
Swift, on the other hand, is perfect for Apple-centric environments. It’s type-safe, compiles fast, and fits iOS or macOS workflows like a glove. You can run Swift on AWS Lambda these days too, but it’s still less common than Node.
In short:
- Node if you want broad cloud support, lots of packages, or you already have JavaScript devs.
- Swift if you’re all-in on Apple platforms and want that performance and strict typing.
Read more: Node.js vs. Swift: Technology Comparison
8. Best practices and things to watch out for
Serverless is awesome, but not without pitfalls:
8.1 Observability and debugging
Logs end up in different places. Use some sort of tracing or centralized logging (like CloudWatch, DataDog, etc.) so you can see the bigger picture.
8.2 Security and permissions
Give each function the least access possible. Don’t let a function that updates one table have admin rights to the entire DB. Lock down secrets and keys.
8.3 Vendor lock-in
Providers do triggers and logs differently. If you lean heavily on AWS Step Functions, moving to Azure gets messy. Tools like Serverless Framework or Terraform help, but there’s no perfect fix.
8.4 Cold starts
If your function doesn’t run often, the first call may be slow. You can keep it warm, but that costs money and makes your setup a bit more complex.
9. Challenges in debugging and distributed tracing
Functions are stateless and often short-lived. Local tests won’t always mimic real traffic or concurrency. Logging and tracing become your best friends. Tag everything, use request IDs, and consider canary releases to test changes on a small chunk of traffic before going all-in.
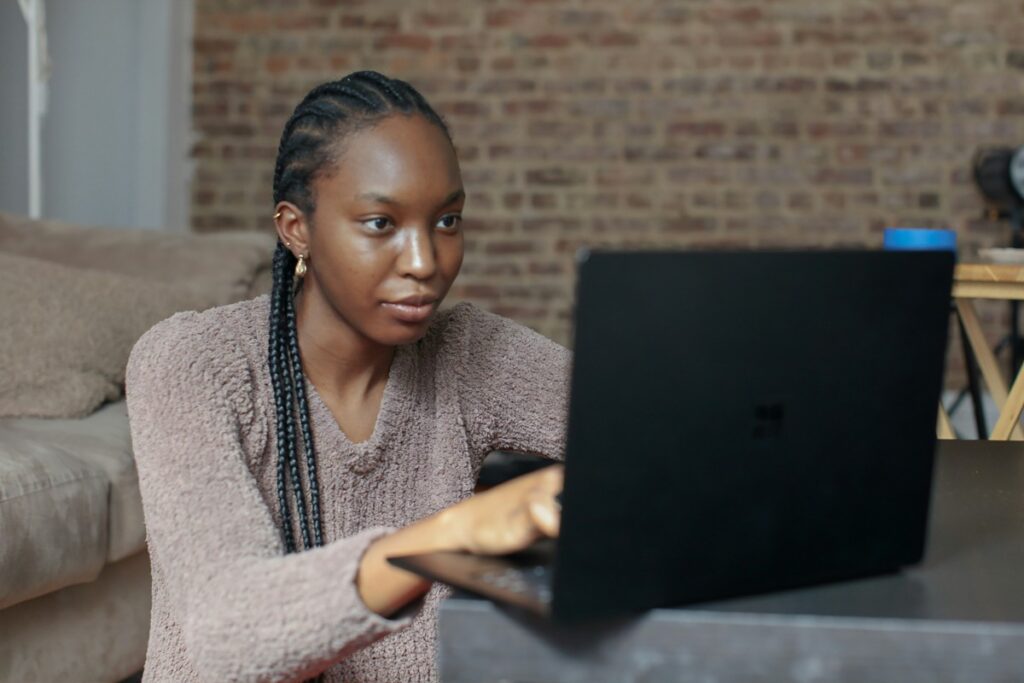
10. Broader benefits of serverless adoption
Why teams go serverless
- Lower costs — no idle servers to pay for
- Faster dev cycles — focus on features, not machine configs
- Built-in scaling — traffic bursts don’t break anything
Real-world use cases
- Webhooks & APIs — easy event handlers for form submissions or external calls
- Data pipelines — triggered code that cleans or moves data
- Chatbots — hooking into NLP services in real time
- IoT streams — handle device data bursts without provisioning huge clusters
11. Real-world illustration
Think of an online store. Someone uploads a product photo, and a function resizes it and stores it. That’s it. No always-on server. You pay only when someone actually uploads a new image.
Same with user sign-ins. You don’t need a server waiting around. A function runs when someone tries to log in, checks their info, then shuts down.
IoT works similarly. Maybe devices send data in bursts, maybe it’s quiet at night. A queue picks up the data, and when it piles up, functions spin up to process it. When things go idle, no cost.
It’s all about paying for what you use. No more, no less.
12. Strategic deployment considerations
Large serverless projects aren’t a free ride. You still have to plan:
- Event triggers — which events set off which function?
- Runtime choice — Node, Swift, Python, Go… pick what your team knows and what your workload needs.
- Security — minimal permissions, encrypted secrets, proper IAM roles.
- Deployment tools — frameworks like Serverless Framework or AWS SAM help with versioning and updates.
- Load testing — see how it behaves under stress, adjust memory or concurrency as needed.
13. Concluding observations and future outlook
Serverless rethinks infrastructure. You’re not running a server 24/7, you’re running code when it’s needed. That saves money, scales easier, and takes less elbow grease to maintain. Still, it’s not a cure-all: debugging can be tricky, cold starts can slow you down, and heavy reliance on a single provider might box you in.
But overall, providers are improving these downsides. Better orchestration, shorter cold starts, and more language support mean serverless can tackle bigger, more complex apps than it used to. If you want a closer look at how everything fits together, a Serverless Architecture Deep Dive will show you the nuts and bolts of it all.
If you’ve got solid logging, security, and architecture, you can build systems that adapt fast and cost less. You’re not stuck watching server metrics or babysitting hardware — you can focus on the code that matters.
In the end, serverless doesn’t mean servers disappear. They’re still there — just hidden away and managed by someone else.